Recommended Pre-Requisites- HTML, CSS, JS
Javascript ES6 Concepts like const and let, arrow functions, template literals, default parameter, array and object de-structuring, array methods, object methods, import-export, promises, async and await(simplified version of promises), spread operator(simplified syntax to copy elements of array or object), classes.
Introduction to React - What is React? Why React? React version history, React 16 vs React 15, Just React – Hello World, Using create-react-app, Anatomy of react project, Running the app, Debugging first react app
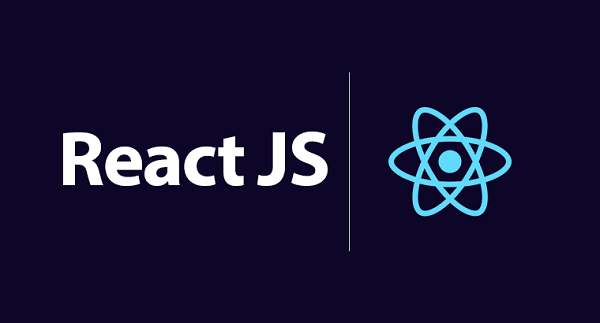
Basic Concepts -
1. Introducing JSX (Javascript-XML like syntax)- Working with React. createElement,
Expressions,
Using logical operators,
Specifying attributes,
Specifying children,
Fragments
2. Components - Significance of component architecture,
Types of components,
Functional,
Class based,
Pure,
Component Composition
3. State and Props - What is state and it significance,
Read state and set state,
Passing data to component using props,
Validating props using propTypes,
Supplying default values to props using defaultProps
4. Rendering Lists
5. Handling Events - Understanding React event system,
Understanding Synthetic event,
Passing arguments to event handlers
6. Understanding component lifecycle and handling errors
7. Conditional Rendering
8. Lists and Keys
9. Lifting State Up
10. React Forms - Controlled components,
Uncontrolled components,
Understand the significance to default Value prop,
Using react ref prop to get access to DOM element
11. Composition and Inheritance
Advanced Concepts -
1. Code-Splitting - What is code splitting,
Why do you need code splitting,
React.lazy,
Suspense,
Route-based code splitting
2. Context API - What is context,
When to use context,
Create Context,
Context.Provider,
Context.Consumer,
Reading context in class
3. Error Boundaries
4. Forwarding Refs
5. Fragments
6. Higher-Order Components
7. Integrating with third-party libraries
Hooks -
1. Introduction
2. Different types of react hooks- State hook, Effect hook, etc.
3. Building your own hooks
Integrating API’s in React
Redux - What is redux, Why redux, Redux principles, Install and setup redux, Creating actions, reducer and store, What is React Redux, Why React Redux, Install and setup, Presentational vs Container components, Understand high order component, Understanding mapStateToProps and mapDispatchtToProps usage